libsimdpp
0.9.3
|
Functions | |
float32x4 | simdpp::abs (float32x4 a) |
Computes absolute value of floating point values. More... | |
float32x8 | simdpp::abs (float32x8 a) |
mask_float32x4 | simdpp::isnan (float32x4 a) |
Checks whether elements in a are IEEE754 NaN. More... | |
mask_float32x8 | simdpp::isnan (float32x8 a) |
Checks whether elements in a are IEEE754 NaN. More... | |
float64x2 | simdpp::abs (float64x2 a) |
Computes absolute value of floating point values. More... | |
float64x4 | simdpp::abs (float64x4 a) |
Computes absolute value of floating point values. More... | |
float32x4 | simdpp::sign (float32x4 a) |
Extracts sign bits from the values in float32x4 vector. More... | |
float32x8 | simdpp::sign (float32x8 a) |
Extracts sign bits from the values in float32x4 vector. More... | |
float64x2 | simdpp::sign (float64x2 a) |
Extracts sigh bit from the values in float64x2 vector. More... | |
float64x4 | simdpp::sign (float64x4 a) |
Extracts sigh bit from the values in float64x2 vector. More... | |
float32x4 | simdpp::add (float32x4 a, float32x4 b) |
Adds the values of two vectors. More... | |
float32x8 | simdpp::add (float32x8 a, float32x8 b) |
Adds the values of two vectors. More... | |
float64x2 | simdpp::add (float64x2 a, float64x2 b) |
Adds the values of two vectors. More... | |
float64x4 | simdpp::add (float64x4 a, float64x4 b) |
Adds the values of two vectors. More... | |
float32x4 | simdpp::sub (float32x4 a, float32x4 b) |
Substracts the values of two vectors. More... | |
float32x8 | simdpp::sub (float32x8 a, float32x8 b) |
Substracts the values of two vectors. More... | |
float64x2 | simdpp::sub (float64x2 a, float64x2 b) |
Subtracts the values of two vectors. More... | |
float64x4 | simdpp::sub (float64x4 a, float64x4 b) |
Subtracts the values of two vectors. More... | |
float32x4 | simdpp::neg (float32x4 a) |
Negates the values of a float32x4 vector. More... | |
float32x8 | simdpp::neg (float32x8 a) |
Negates the values of a float32x4 vector. More... | |
float64x2 | simdpp::neg (float64x2 a) |
Negates the values of a vector. More... | |
float64x4 | simdpp::neg (float64x4 a) |
Negates the values of a vector. More... | |
float32x4 | simdpp::mul (float32x4 a, float32x4 b) |
Multiplies the values of two vectors. More... | |
float32x8 | simdpp::mul (float32x8 a, float32x8 b) |
Multiplies the values of two vectors. More... | |
float64x2 | simdpp::mul (float64x2 a, float64x2 b) |
Multiplies the values of two vectors. More... | |
float64x4 | simdpp::mul (float64x4 a, float64x4 b) |
Multiplies the values of two vectors. More... | |
float32x4 | simdpp::fmadd (float32x4 a, float32x4 b, float32x4 c) |
Performs a fused multiply-add operation. More... | |
float32x8 | simdpp::fmadd (float32x8 a, float32x8 b, float32x8 c) |
Performs a fused multiply-add operation. More... | |
float64x2 | simdpp::fmadd (float64x2 a, float64x2 b, float64x2 c) |
Performs a fused multiply-add operation. More... | |
float64x4 | simdpp::fmadd (float64x4 a, float64x4 b, float64x4 c) |
Performs a fused multiply-add operation. More... | |
float32x4 | simdpp::fmsub (float32x4 a, float32x4 b, float32x4 c) |
Performs a fused multiply-sutract operation. More... | |
float32x8 | simdpp::fmsub (float32x8 a, float32x8 b, float32x8 c) |
Performs a fused multiply-sutract operation. More... | |
float64x2 | simdpp::fmsub (float64x2 a, float64x2 b, float64x2 c) |
Performs a fused multiply-sutract operation. More... | |
float64x4 | simdpp::fmsub (float64x4 a, float64x4 b, float64x4 c) |
Performs a fused multiply-sutract operation. More... | |
Detailed Description
Function Documentation
|
inline |
Computes absolute value of floating point values.
- 128-bit version:
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- In ALTIVEC this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In NEON this intrinsic results in at least 2 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
- In ALTIVEC this intrinsic results in at least 2-3 instructions.
|
inline |
|
inline |
Computes absolute value of floating point values.
- 128-bit version:
- Not vectorized in NEON and .
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
|
inline |
Computes absolute value of floating point values.
- 128-bit version:
- Not vectorized in NEON and .
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
|
inline |
Adds the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Adds the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Adds the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
|
inline |
Adds the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
|
inline |
Performs a fused multiply-add operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-add operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-add operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-add operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-sutract operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-sutract operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-sutract operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
Performs a fused multiply-sutract operation.
Implemented only on architectures with either X86_FMA3
or X86_FMA4
support.
|
inline |
|
inline |
|
inline |
Multiplies the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Multiplies the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Multiplies the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
|
inline |
Multiplies the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
|
inline |
Negates the values of a float32x4 vector.
- 128-bit version:
- In SSE2-AVX2 and ALTIVEC this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- In SSE2-SSE4.1 and ALTIVEC this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 and NEON this intrinsic results in at least 2 instructions.
|
inline |
Negates the values of a float32x4 vector.
- 128-bit version:
- In SSE2-AVX2 and ALTIVEC this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- In SSE2-SSE4.1 and ALTIVEC this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 and NEON this intrinsic results in at least 2 instructions.
|
inline |
Negates the values of a vector.
- 128-bit version:
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
- 256-bit version:
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
|
inline |
Negates the values of a vector.
- 128-bit version:
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
- 256-bit version:
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
|
inline |
Extracts sign bits from the values in float32x4 vector.
- 128-bit version:
- In SSE2-SSE4.1, ALTIVEC and NEON this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- In SSE2-SSE4.1, ALTIVEC and NEON this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
|
inline |
Extracts sign bits from the values in float32x4 vector.
- 128-bit version:
- In SSE2-SSE4.1, ALTIVEC and NEON this intrinsic results in at least 1-2 instructions.
- 256-bit version:
- In SSE2-SSE4.1, ALTIVEC and NEON this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
|
inline |
Extracts sigh bit from the values in float64x2 vector.
- 128-bit version:
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
- 256-bit version:
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
|
inline |
Extracts sigh bit from the values in float64x2 vector.
- 128-bit version:
- In SSE2-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
- 256-bit version:
- In SSE2-SSE4.1 this intrinsic results in at least 2-3 instructions.
- In AVX-AVX2 this intrinsic results in at least 1-2 instructions.
- Not vectorized in NEON and .
|
inline |
Substracts the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Substracts the values of two vectors.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Subtracts the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
|
inline |
Subtracts the values of two vectors.
- 128-bit version:
- Not vectorized in NEON and .
- 256-bit version:
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 2 instructions.
Generated on Thu Oct 31 2013 04:08:51 for libsimdpp by
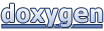