libsimdpp
0.9.3
|
Functions | |
template<class R , class T > | |
R | simdpp::bit_cast (T t) |
Casts between unrelated types. More... | |
basic_int16x8 | simdpp::to_int16x8 (int8x16 a) |
Sign extends the first 8 values of a signed int8x16 vector to 16-bits. More... | |
basic_int16x16 | simdpp::to_int16x16 (int8x32 a) |
Sign extends the first 16 values of a signed int8x32 vector to 16-bits. More... | |
basic_int16x8 | simdpp::to_int16x8 (uint8x16 a) |
Extends the first 8 values of a unsigned int8x16 vector to 16-bits. More... | |
basic_int16x16 | simdpp::to_int16x16 (uint8x32 a) |
Extends the first 16 values of a unsigned int8x32 vector to 16-bits. More... | |
basic_int32x4 | simdpp::to_int32x4 (int16x8 a) |
Sign extends the first 4 values of a signed int16x8 vector to 32-bits. More... | |
basic_int32x8 | simdpp::to_int32x8 (int16x16 a) |
Sign extends the first 8 values of a signed int16x16 vector to 32-bits. More... | |
basic_int32x4 | simdpp::to_int32x4 (uint16x8 a) |
Zero-extends the values of a unsigned int16x8 vector to 32-bits. More... | |
basic_int32x8 | simdpp::to_int32x8 (uint16x16 a) |
Zero-extends the first 8 values of a unsigned int16x16 vector to 32-bits. More... | |
basic_int32x4 | simdpp::to_int32x4 (float32x4 a) |
Converts the values of a float32x4 vector into signed int32_t representation using truncation if only an inexact conversion can be performed. More... | |
basic_int32x8 | simdpp::to_int32x8 (float32x8 a) |
Converts the values of a float32x4 vector into signed int32_t representation using truncation if only an inexact conversion can be performed. More... | |
basic_int32x4 | simdpp::to_int32x4 (float64x2 a) |
Converts the values of a doublex2 vector into int32_t representation using truncation. More... | |
basic_int32x8 | simdpp::to_int32x8 (float64x4 a) |
Converts the values of a doublex2 vector into int32_t representation using truncation. More... | |
basic_int64x2 | simdpp::to_int64x2 (int32x4 a) |
Extends the values of a signed int32x4 vector to 64-bits. More... | |
basic_int64x4 | simdpp::to_int64x4 (int32x8 a) |
Extends the values of a signed int32x4 vector to 64-bits. More... | |
basic_int64x2 | simdpp::to_int64x2 (uint32x4 a) |
Extends the values of an unsigned int32x4 vector to 64-bits. More... | |
basic_int64x4 | simdpp::to_int64x4 (uint32x8 a) |
Extends the values of a signed int32x4 vector to 64-bits. More... | |
float32x4 | simdpp::to_float32x4 (int32x4 a) |
Converts 32-bit integer values to 32-bit float values. More... | |
float32x8 | simdpp::to_float32x8 (int32x8 a) |
Converts 32-bit integer values to 32-bit float values. More... | |
float32x4 | simdpp::to_float32x4 (float64x2 a) |
Converts 64-bit float values to 32-bit float values. More... | |
float32x8 | simdpp::to_float32x8 (float64x4 a) |
Converts 64-bit float values to 32-bit float values. More... | |
float64x2 | simdpp::to_float64x2 (int32x4 a) |
Converts the 32-bit integer values to 64-bit float values. More... | |
float64x4 | simdpp::to_float64x4 (int32x8 a) |
Converts the 32-bit integer values to 64-bit float values. More... | |
float64x2 | simdpp::to_float64x2 (float32x4 a) |
Converts the 32-bit float values to 64-bit float values. More... | |
float64x4 | simdpp::to_float64x4 (float32x8 a) |
Converts the 32-bit float values to 64-bit float values. More... | |
Detailed Description
Function Documentation
R simdpp::bit_cast | ( | T | t | ) |
Casts between unrelated types.
No changes to the stored values are performed.
|
inline |
Converts 32-bit integer values to 32-bit float values.
SSE specific:
If only inexact conversion can be performed, the current rounding mode is used.
NEON, ALTIVEC specific:
If only inexact conversion can be performed, round to nearest mode is used.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Converts 64-bit float values to 32-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is truncated.
- 128-bit version:
- r0 = (float) a0r1 = (float) a1r2 = 0.0fr3 = 0.0f
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (float) a0...r3 = (float) a3r4 = 0.0f...r7 = 0.0f
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Converts 32-bit integer values to 32-bit float values.
SSE specific:
If only inexact conversion can be performed, the current rounding mode is used.
NEON, ALTIVEC specific:
If only inexact conversion can be performed, round to nearest mode is used.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Converts 64-bit float values to 32-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is truncated.
- 128-bit version:
- r0 = (float) a0r1 = (float) a1r2 = 0.0fr3 = 0.0f
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (float) a0...r3 = (float) a3r4 = 0.0f...r7 = 0.0f
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Converts the 32-bit integer values to 64-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is rounded to the nearest representable value.
- 128-bit version:
- r0 = (double) a0r1 = (double) a1
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (double) a0...r3 = (double) a3
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Converts the 32-bit float values to 64-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is rounded to the nearest representable value.
- 128-bit version:
- r0 = (double) a0r1 = (double) a1
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (double) a0...r3 = (double) a3
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Converts the 32-bit integer values to 64-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is rounded to the nearest representable value.
- 128-bit version:
- r0 = (double) a0r1 = (double) a1
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (double) a0...r3 = (double) a3
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Converts the 32-bit float values to 64-bit float values.
SSE specific:
If only inexact conversion can be performed, the value is rounded according to the current rounding mode.
NEON specific:
If only inexact conversion can be performed, the value is rounded to the nearest representable value.
- 128-bit version:
- r0 = (double) a0r1 = (double) a1
- Not vectorized in NEON and .
- 256-bit version:
- r0 = (double) a0...r3 = (double) a3
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
- Not vectorized in NEON and .
|
inline |
Sign extends the first 16 values of a signed int8x32 vector to 16-bits.
- In SSE4.1-AVX this intrinsic results in at least 3 instructions.
- In SSE2-SSSE3 this intrinsic results in at least 4 instructions.
- In NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Extends the first 16 values of a unsigned int8x32 vector to 16-bits.
- In SSE2-AVX, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Sign extends the first 8 values of a signed int8x16 vector to 16-bits.
- In SSE2-SSSE3 this intrinsic results in at least 2 instructions.
|
inline |
Extends the first 8 values of a unsigned int8x16 vector to 16-bits.
|
inline |
Sign extends the first 4 values of a signed int16x8 vector to 32-bits.
- In SSE2-SSSE3 this intrinsic results in at least 2 instructions.
|
inline |
Zero-extends the values of a unsigned int16x8 vector to 32-bits.
|
inline |
Converts the values of a float32x4 vector into signed int32_t representation using truncation if only an inexact conversion can be performed.
The behavior is undefined if the value can not be represented in the result type.
SSE specific: If the value can not be represented by int32_t, 0x80000000
is returned TODO: NaN handling
NEON, ALTIVEC specific: If the value can not be represented by int32_t, either 0x80000000
or 0x7fffffff
is returned depending on the sign of the operand (saturation occurs). Conversion of NaNs results in 0.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Converts the values of a doublex2 vector into int32_t representation using truncation.
The behavior is undefined if the value can not be represented in the result type.
SSE specific: If the value can not be represented by int32_t, 0x80000000
is returned
- Todo:
- NaN handling
NEON VFP specific: If the value can not be represented by int32_t, either 0x80000000
or 0x7fffffff
is returned depending on the sign of the operand. Conversion of NaNs results in 0.
- 128-bit version:
- Not vectorized in NEON and .
r0 = (int32_t) a0r1 = (int32_t) a1r2 = 0r3 = 0
- 256-bit version:
- r0 = (int32_t) a0...r3 = (int32_t) a3r4 = 0...r7 = 0
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
|
inline |
Sign extends the first 8 values of a signed int16x16 vector to 32-bits.
- In SSE4.1-AVX this intrinsic results in at least 3 instructions.
- In SSE2-SSSE3 this intrinsic results in at least 4 instructions.
- In NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Zero-extends the first 8 values of a unsigned int16x16 vector to 32-bits.
- In SSE2-AVX and ALTIVEC this intrinsic results in at least 2 instructions.
- In NEON this intrinsic results in at least 2 instructions.
|
inline |
Converts the values of a float32x4 vector into signed int32_t representation using truncation if only an inexact conversion can be performed.
The behavior is undefined if the value can not be represented in the result type.
SSE specific: If the value can not be represented by int32_t, 0x80000000
is returned TODO: NaN handling
NEON, ALTIVEC specific: If the value can not be represented by int32_t, either 0x80000000
or 0x7fffffff
is returned depending on the sign of the operand (saturation occurs). Conversion of NaNs results in 0.
- 256-bit version:
- In SSE2-SSE4.1, NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Converts the values of a doublex2 vector into int32_t representation using truncation.
The behavior is undefined if the value can not be represented in the result type.
SSE specific: If the value can not be represented by int32_t, 0x80000000
is returned
- Todo:
- NaN handling
NEON VFP specific: If the value can not be represented by int32_t, either 0x80000000
or 0x7fffffff
is returned depending on the sign of the operand. Conversion of NaNs results in 0.
- 128-bit version:
- Not vectorized in NEON and .
r0 = (int32_t) a0r1 = (int32_t) a1r2 = 0r3 = 0
- 256-bit version:
- r0 = (int32_t) a0...r3 = (int32_t) a3r4 = 0...r7 = 0
- Not vectorized in NEON and .
- In SSE2-SSE4.1 this intrinsic results in at least 3 instructions.
|
inline |
Extends the values of a signed int32x4 vector to 64-bits.
- 128-bit version:
- r0 = (int64_t) a0r1 = (int64_t) a1
- In SSE2-SSSE3 this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 2-3 instructions.
- 256-bit version:
- r0 = (int64_t) a0...r3 = (int64_t) a3
- In SSE2-SSSE3 this intrinsic results in at least 5 instructions.
- In SSE4.1-AVX this intrinsic results in at least 3 instructions.
- In NEON this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 3-4 instructions.
|
inline |
Extends the values of an unsigned int32x4 vector to 64-bits.
- 128-bit version:
- r0 = (uint64_t) a0r1 = (uint64_t) a1
- 256-bit version:
- r0 = (uint64_t) a0...r3 = (uint64_t) a3
- In SSE2-AVX this intrinsic results in at least 3 instructions.
- In NEON and ALTIVEC this intrinsic results in at least 2 instructions.
|
inline |
Extends the values of a signed int32x4 vector to 64-bits.
- 128-bit version:
- r0 = (int64_t) a0r1 = (int64_t) a1
- In SSE2-SSSE3 this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 2-3 instructions.
- 256-bit version:
- r0 = (int64_t) a0...r3 = (int64_t) a3
- In SSE2-SSSE3 this intrinsic results in at least 5 instructions.
- In SSE4.1-AVX this intrinsic results in at least 3 instructions.
- In NEON this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 3-4 instructions.
|
inline |
Extends the values of a signed int32x4 vector to 64-bits.
- 128-bit version:
- r0 = (int64_t) a0r1 = (int64_t) a1
- In SSE2-SSSE3 this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 2-3 instructions.
- 256-bit version:
- r0 = (int64_t) a0...r3 = (int64_t) a3
- In SSE2-SSSE3 this intrinsic results in at least 5 instructions.
- In SSE4.1-AVX this intrinsic results in at least 3 instructions.
- In NEON this intrinsic results in at least 2 instructions.
- In ALTIVEC this intrinsic results in at least 3-4 instructions.
Generated on Thu Oct 31 2013 04:08:50 for libsimdpp by
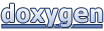