libsimdpp
0.9.3
|
Class representing 16x 8-bit unsigned integer vector. More...
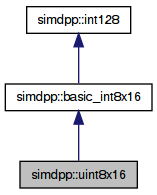
Public Member Functions | |
uint8x16 () | |
uint8x16 (const uint8x16 &d) | |
uint8x16 & | operator= (const uint8x16 &d) |
uint8x16 (const int128 &d) | |
uint8x16 (const basic_int8x16 &d) | |
uint8x16 & | operator= (int128 d) |
uint8x16 & | operator= (basic_int8x16 d) |
![]() | |
basic_int8x16 () | |
basic_int8x16 (const basic_int8x16 &d) | |
basic_int8x16 & | operator= (const basic_int8x16 &d) |
basic_int8x16 (const int128 &d) | |
basic_int8x16 & | operator= (const int128 &d) |
![]() | |
int128 () | |
int128 (const int128 &d) | |
int128 & | operator= (const int128 &d) |
Static Public Member Functions | |
static uint8x16 | zero () |
Creates a unsigned int8x16 vector with the contents set to zero. More... | |
static uint8x16 | load_broadcast (const uint8_t *v0) |
Creates a unsigned int8x16 vector from a value loaded from memory. More... | |
static uint8x16 | set_broadcast (uint8_t v0) |
Creates a unsigned int8x16 vector from a value stored in a core register. More... | |
static uint8x16 | make_const (uint8_t v0) |
Creates a unsigned int8x16 vector from a value known at compile-time. More... | |
static uint8x16 | make_const (uint8_t v0, uint8_t v1) |
Creates a unsigned int8x16 vector from two values known at compile-time. More... | |
static uint8x16 | make_const (uint8_t v0, uint8_t v1, uint8_t v2, uint8_t v3) |
Creates a unsigned int8x16 vector from four values known at compile-time. More... | |
static uint8x16 | make_const (uint8_t v0, uint8_t v1, uint8_t v2, uint8_t v3, uint8_t v4, uint8_t v5, uint8_t v6, uint8_t v7) |
Creates a unsigned int8x16 vector from eight values known at compile-time. More... | |
static uint8x16 | make_const (uint8_t v0, uint8_t v1, uint8_t v2, uint8_t v3, uint8_t v4, uint8_t v5, uint8_t v6, uint8_t v7, uint8_t v8, uint8_t v9, uint8_t v10, uint8_t v11, uint8_t v12, uint8_t v13, uint8_t v14, uint8_t v15) |
Creates a unsigned int8x16 vector from sixteen values known at compile-time. More... | |
Additional Inherited Members | |
![]() | |
typedef uint8_t | element_type |
typedef uint8_t | uint_element_type |
typedef basic_int8x16 | int_vector_type |
typedef uint8x16 | uint_vector_type |
typedef mask_int8x16 | mask_type |
![]() | |
static const unsigned | length = 16 |
static const unsigned | num_bits = 8 |
static const uint_element_type | all_bits = 0xff |
Detailed Description
Class representing 16x 8-bit unsigned integer vector.
Constructor & Destructor Documentation
|
inline |
|
inline |
|
inline |
Construct from the underlying vector type Construct from the base type
|
inline |
Construct from the underlying vector type Construct from the base type
Member Function Documentation
|
static |
Creates a unsigned int8x16 vector from a value loaded from memory.
- In SSE2-SSE4.1 this intrinsic results in at least 4 instructions.
- In NEON this intrinsic results in at least 1 instructions.
|
static |
Creates a unsigned int8x16 vector from a value known at compile-time.
|
static |
Creates a unsigned int8x16 vector from two values known at compile-time.
|
static |
Creates a unsigned int8x16 vector from four values known at compile-time.
|
static |
Creates a unsigned int8x16 vector from eight values known at compile-time.
|
static |
Creates a unsigned int8x16 vector from sixteen values known at compile-time.
Construct from the underlying vector type Construct from the base type
|
inline |
Construct from the underlying vector type Construct from the base type
|
static |
Creates a unsigned int8x16 vector from a value stored in a core register.
- In SSE2-SSE4.1 and NEON this intrinsic results in at least 3 instructions.
|
static |
Creates a unsigned int8x16 vector with the contents set to zero.
The documentation for this class was generated from the following file:
- simd/int8x16.h
Generated on Thu Oct 31 2013 04:08:52 for libsimdpp by
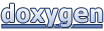